In the world of modern web development, mastering state management is crucial for building robust applications. Redux has emerged as a popular library for managing application state efficiently, and understanding how to effectively implement a Redux build is essential for developers. This article will explore the intricacies of Redux builds, providing insights into its architecture, benefits, and best practices.
Redux allows developers to maintain a predictable state container, making it easier to manage application data. Whether you are building a small-scale application or a large enterprise-level project, knowing how to create a Redux build can significantly improve your development workflow. In this guide, we will delve into the key concepts of Redux, the process of setting up a Redux build, and the advantages it offers for application development.
This article is designed for both beginners and experienced developers seeking to enhance their knowledge of Redux. By the end of this comprehensive guide, you will have a clear understanding of Redux builds and how they can streamline your application development process.
Table of Contents
- What is Redux?
- Redux Architecture
- Setting Up a Redux Build
- Core Concepts of Redux
- Advanced Features of Redux
- Benefits of Using Redux
- Common Mistakes in Redux Builds
- Best Practices for Redux Builds
What is Redux?
Redux is a predictable state container for JavaScript applications. It is commonly used with libraries like React for building user interfaces. Redux provides a centralized store that holds the application state, allowing components to access and modify that state in a predictable manner.
By using Redux, developers can manage the application state more efficiently, making it easier to debug and test applications. The core principle of Redux is that the entire application state is stored in a single object, which can be accessed and updated through actions and reducers.
Key Features of Redux
- Single Source of Truth: The entire state of the application is stored in one place.
- State is Read-Only: The only way to change the state is by dispatching actions.
- Changes are Made with Pure Functions: Reducers are pure functions that take the previous state and an action to return the next state.
Redux Architecture
The architecture of Redux revolves around three core components: actions, reducers, and the store. Understanding how these components interact is key to mastering Redux builds.
Actions
Actions are plain JavaScript objects that represent an intention to change the state. Each action must have a type property that indicates the type of action being performed. Actions can also include additional data needed to update the state.
Reducers
Reducers are pure functions that take the current state and an action as arguments and return a new state. They determine how the state should change in response to an action.
Store
The store is the central hub that holds the application state. It allows access to the state via `getState()`, updates the state via `dispatch(action)`, and registers listeners via `subscribe(listener)`.
Setting Up a Redux Build
To set up a Redux build, you need to follow a series of steps, including installing the necessary packages, configuring the store, and creating actions and reducers. Below is a step-by-step guide.
Step 1: Install Redux
Start by installing Redux and React-Redux in your project using npm:
npm install redux react-redux
Step 2: Create the Redux Store
Next, create a store by importing `createStore` from Redux and passing in your root reducer:
import { createStore } from 'redux'; import rootReducer from './reducers'; const store = createStore(rootReducer);
Step 3: Provide the Redux Store
Wrap your main application component with the `Provider` component from React-Redux to make the store available to all components:
import { Provider } from 'react-redux';
Core Concepts of Redux
Understanding the core concepts of Redux is essential for building effective applications. Here are some fundamental concepts to grasp:
Middleware
Middleware in Redux allows you to extend Redux with custom functionality, such as logging actions or handling asynchronous operations. Common middleware includes Redux Thunk and Redux Saga.
Selectors
Selectores are functions that extract specific pieces of data from the Redux store. They help to encapsulate the logic for accessing state, making it easier to manage and test.
Advanced Features of Redux
Once you are comfortable with the basics of Redux, you can explore advanced features that enhance its functionality:
Redux Toolkit
Redux Toolkit is the official recommended way to write Redux logic. It provides a set of tools and best practices to streamline the Redux development process.
Reselect
Reselect is a library that allows you to create memoized selectors, improving performance by preventing unnecessary re-computations of derived data.
Benefits of Using Redux
Utilizing Redux in your projects comes with several advantages:
- Predictable State Management: Redux ensures that state changes are predictable and can be traced easily.
- Improved Debugging: Tools like Redux DevTools enable developers to inspect every action and state change.
- Enhanced Collaboration: The clear architecture of Redux facilitates collaboration among developers.
Common Mistakes in Redux Builds
When working with Redux, developers often make several common mistakes that can hinder application performance:
- Not Normalizing State: Avoid deeply nested state objects to simplify the state structure.
- Overusing Redux: Not every piece of state needs to be in Redux; use it judiciously.
- Ignoring Performance Optimization: Utilize memoization and selectors to improve performance.
Best Practices for Redux Builds
To make the most of Redux, consider the following best practices:
- Keep Reducers Pure: Ensure that reducers do not have side effects and are purely functional.
- Use Redux Toolkit: Leverage the Redux Toolkit for a simplified development process.
- Organize Code: Structure your Redux code into separate folders for actions, reducers, and selectors.
Conclusion
In conclusion, understanding Redux builds is essential for any developer looking to manage state effectively in their applications. By mastering the core concepts of Redux, setting up a proper build, and following best practices, you can create scalable and maintainable applications.
We encourage you to share your thoughts in the comments below, explore further articles on our site, and continue your journey in mastering Redux and state management.
Closing Thoughts
Thank you for reading! We hope this comprehensive guide on Redux builds has provided valuable insights and knowledge. Stay tuned for more articles that delve into the world of web development and state management.
Article Recommendations
- Hig Roberts
- Dinosaur Dung
- Debutante
- Mia Hamm Soccer Player
- Kamila Valieva
- Global Impact_0.xml
- Future Opportunities_0.xml
- Non Fiction Meaning
- Ella Bleu S Career Updates
- Creative Solutions_0.xml

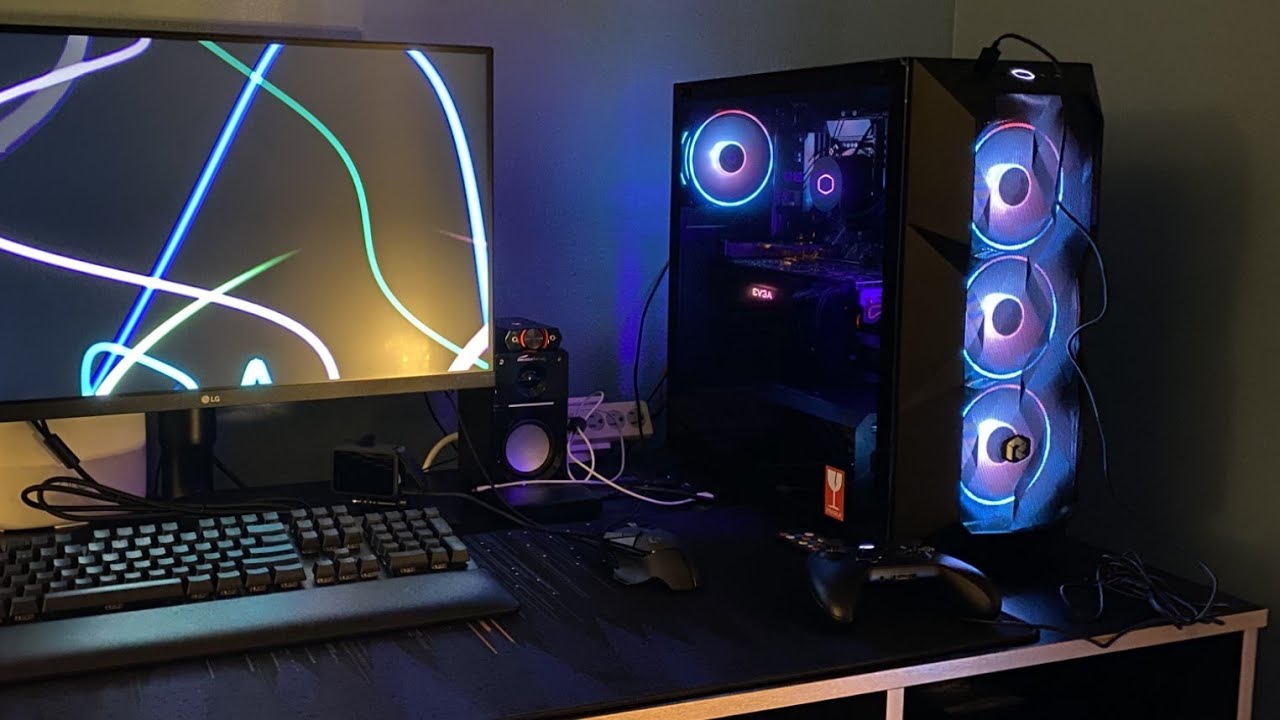
