NPM Testing is an essential aspect of modern software development, particularly in the JavaScript ecosystem. As developers strive for high-quality code, understanding how to effectively test applications using NPM (Node Package Manager) becomes crucial. This guide will provide an in-depth look at NPM testing, including its importance, tools, methodologies, and best practices.
With the rapid evolution of software development practices, testing has become more than just a necessity; it is a fundamental part of the development lifecycle. NPM testing not only helps in identifying bugs but also ensures that the application performs as expected in various scenarios. In this article, we will explore various testing frameworks, how to set them up, and the best practices to maintain quality code.
Whether you are a seasoned developer or just starting out, understanding NPM testing can significantly enhance your coding skills and the overall quality of your projects. By the end of this article, you will have a clear understanding of how to implement effective testing strategies using NPM.
Table of Contents
- What is NPM Testing?
- Importance of NPM Testing
- Popular NPM Testing Frameworks
- Setting Up a Testing Environment
- Types of Testing with NPM
- Best Practices for NPM Testing
- Common Mistakes to Avoid in NPM Testing
- Conclusion and Next Steps
What is NPM Testing?
NPM testing refers to the process of testing JavaScript applications using the Node Package Manager and its associated libraries and tools. It involves writing tests to verify that the code behaves as expected, ensuring that any changes or new features do not break existing functionality.
The primary goal of NPM testing is to catch bugs early in the development process, which can save time and resources in the long run. There are various types of testing that can be performed within the NPM ecosystem, including unit testing, integration testing, and end-to-end testing.
Importance of NPM Testing
Testing is crucial in software development for several reasons:
- Quality Assurance: Ensures that the application meets quality standards and functions as intended.
- Early Bug Detection: Identifies issues before they reach production, reducing the cost of fixing bugs.
- Documentation: Tests serve as documentation for how the code is expected to behave.
- Confidence in Refactoring: Allows developers to make changes with the assurance that existing functionality will not be broken.
Popular NPM Testing Frameworks
There are several popular testing frameworks available for NPM, each with its unique features and benefits:
1. Mocha
Mocha is a flexible testing framework for Node.js that supports asynchronous testing. It allows developers to structure their tests and provides options for reporting and running tests in various environments.
2. Jasmine
Jasmine is a behavior-driven development framework for testing JavaScript code. It is known for its simplicity and ease of use, making it an excellent choice for developers new to testing.
3. Jest
Jest is a popular testing framework developed by Facebook. It is designed for testing React applications but can be used with any JavaScript project. Jest offers a rich set of features, including snapshot testing and built-in mocking capabilities.
4. Cypress
Cypress is an end-to-end testing framework that allows developers to test applications in a real browser environment. It provides powerful tools for testing user interactions and can greatly enhance the testing process.
Setting Up a Testing Environment
To begin testing with NPM, developers need to set up a testing environment. This typically involves the following steps:
- Install Node.js and NPM on your machine.
- Initialize a new Node.js project using
npm init
. - Choose a testing framework and install it using NPM (e.g.,
npm install mocha --save-dev
). - Create a test directory to organize your test files.
- Write your first test and run it using the appropriate command (e.g.,
npm test
).
Types of Testing with NPM
There are several types of testing that can be performed using NPM:
1. Unit Testing
Unit testing focuses on testing individual components or functions in isolation. This type of testing ensures that each part of the application behaves correctly.
2. Integration Testing
Integration testing verifies that different modules or services work together as expected. This type of testing is crucial for identifying issues that may arise when components interact.
3. End-to-End Testing
End-to-end testing simulates real user scenarios to ensure that the application functions correctly from start to finish. This type of testing is essential for validating the overall user experience.
4. Functional Testing
Functional testing focuses on verifying that the application performs its intended functions according to the requirements. It is often performed after unit and integration tests.
Best Practices for NPM Testing
To ensure effective NPM testing, consider the following best practices:
- Write Clear and Concise Tests: Ensure that your tests are easy to read and understand.
- Keep Tests Isolated: Each test should be independent to avoid interference between tests.
- Automate Testing: Use Continuous Integration (CI) tools to automate your testing process.
- Run Tests Frequently: Execute tests regularly throughout the development process to catch issues early.
Common Mistakes to Avoid in NPM Testing
Here are some common pitfalls to avoid when conducting NPM testing:
- Neglecting Edge Cases: Always consider edge cases in your tests to ensure comprehensive coverage.
- Over-Testing: Avoid writing excessive tests for trivial code, which can lead to increased maintenance overhead.
- Ignoring Test Results: Always review and act on test results to improve code quality.
- Not Updating Tests: Update tests as the application evolves to ensure they remain relevant.
Conclusion and Next Steps
NPM testing is a vital part of the software development process that ensures your JavaScript applications are reliable and maintainable. By understanding the various testing frameworks, setting up a proper testing environment, and following best practices, you can significantly enhance the quality of your code.
Now that you have a comprehensive understanding of NPM testing, consider implementing these strategies in your projects. Don't hesitate to leave a comment below, share this article with your peers, or explore our other resources on software development!
Thank you for reading, and we hope to see you back for more insightful articles on software development!
Article Recommendations
- Three Cornered Hat Crossword Clue
- Dinosaur Dung
- Madison Beer Nude Leak
- Claudine Blanchard Crimes
- Wallet With Pull Tab
- How To Make Raphael In Infinite Craft
- Digital Revolution_0.xml
- How Tall Sarah Jessica Parker
- Active Smooth Nail Polish
- Lava Stone Bracelet Essential Oil

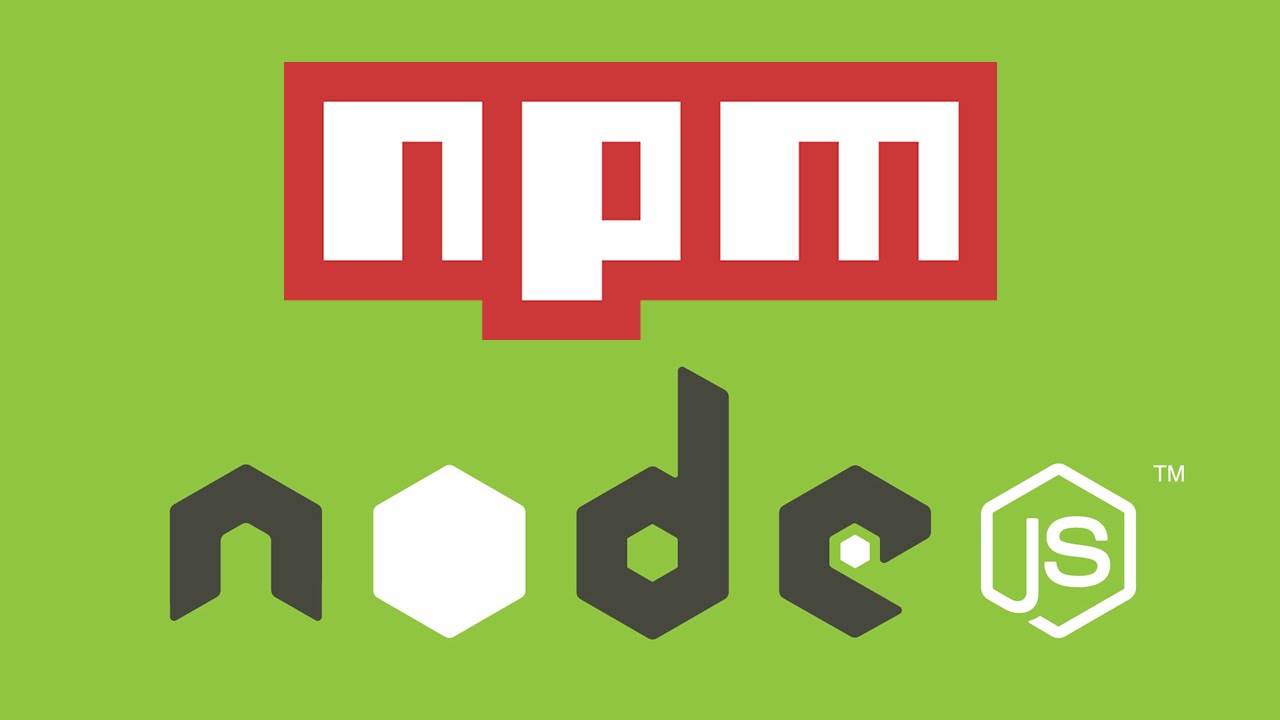