In the realm of programming, understanding how functions operate is fundamental to writing efficient and effective code. One key aspect of functions in Python is the use of the return statement, which plays a crucial role in how data is passed back to the caller after a function execution. This article delves into the intricate details of how to return from a function in Python, exploring its significance, syntax, and various scenarios where it is applicable. By grasping the concept of returning values, developers can enhance their coding skills and create more robust applications.
Functions are reusable pieces of code that perform specific tasks, and the return statement is what allows them to provide output to the code that invoked them. Without this capability, functions would merely execute their operations without any way to communicate results back to the main program. In Python, the return statement can return a single value, multiple values, or even no value at all, depending on the requirements of the program.
This article aims to guide readers through the process of returning from a function in Python, breaking down complex concepts into digestible pieces. We will address common questions, provide examples, and highlight best practices to ensure a comprehensive understanding of this essential programming concept. By the end of this article, you will be equipped with the knowledge to effectively use return statements in your Python functions.
What is the Purpose of Returning from a Function in Python?
Returning from a function in Python allows programmers to extract the result of a computation or operation performed within that function. It serves several purposes:
- To provide output to the caller of the function.
- To enable further processing of the returned value.
- To facilitate modular programming by breaking down complex tasks into smaller, manageable pieces.
- To enhance code readability and maintainability.
How Do You Use the Return Statement in Python?
The return statement is straightforward to use. Below is the basic syntax:
def function_name(parameters): # perform some operation return value
When the return statement is executed, the function terminates, and the specified value is sent back to the caller.
Can a Function Return Multiple Values?
Yes, a function can return multiple values in Python. This is achieved by separating the values with commas in the return statement. For example:
def calculate(a, b): sum = a + b product = a * b return sum, product
When this function is called, it will return both the sum and the product as a tuple.
What Happens If a Function Does Not Return a Value?
If a function does not have a return statement or reaches the end of its block without hitting a return, it implicitly returns None. This can be useful for functions that perform actions but do not need to return any specific value:
def print_message(message): print(message)
In this case, calling the function will display the message, but the return value will be None.
How to Handle Returned Values in Python?
When a function returns a value, it can be captured and used in various ways. Below are some common practices:
- Assigning the return value to a variable:
result = function_name(arguments)
print(function_name(arguments))
sum, product = calculate(5, 3)
What Are Some Best Practices for Using Return from a Function in Python?
To ensure that your functions are efficient and easy to understand, consider the following best practices:
- Always use return statements to provide output when necessary.
- Avoid using multiple return statements in a single function unless it enhances clarity.
- Ensure that your function has a clear purpose and returns values that are relevant to that purpose.
- Document the expected return values in the function's docstring.
How Can You Return Data Structures from a Function?
Python allows you to return complex data structures, such as lists, dictionaries, or custom objects, from a function. For example:
def create_user_info(name, age): return {'name': name, 'age': age}
This function returns a dictionary containing user information, showcasing how versatile Python functions can be.
Conclusion: Mastering Return from a Function in Python
Understanding how to return from a function in Python is a vital skill for any aspiring programmer. By mastering the return statement, you can enhance your coding efficiency, improve code reusability, and ultimately lead to more robust applications. Whether you are returning a single value, multiple values, or working with complex data structures, the principles of returning values from functions are foundational to effective programming in Python. So dive into your coding projects with confidence, knowing that you have the tools to communicate results from your functions effectively!
Article Recommendations
- Corinne Foxx
- Debutante
- Kill Fleas In House
- Light Therapy
- Non Fiction Meaning
- Brand Building_0.xml
- Ne Yo
- Business Resilience_0.xml
- Nikki Minja Naked
- Jennifer Syme Crash
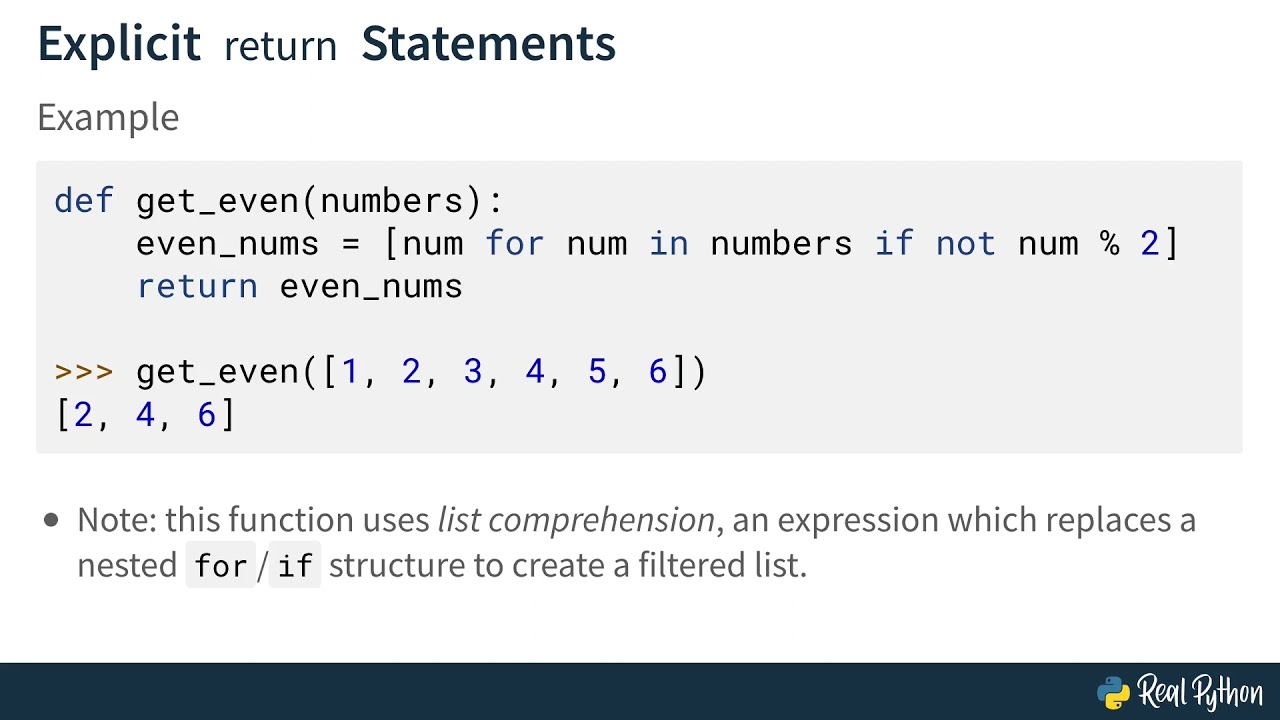

