When it comes to decision-making in programming, the switch case statement in Java stands out as a powerful tool for developers. Unlike the traditional if-else ladder, the switch case construct allows for a more organized and readable approach to handling multiple conditions. It is particularly useful when you have a single variable that needs to be evaluated against a series of possible values. The switch case statement enhances code clarity and reduces the potential for errors, making it an essential feature in the Java programming language.
In Java, the switch case statement can handle primitive types like integers, characters, and strings, making it a versatile choice for various applications. By utilizing switch case, developers can streamline their code, improving maintainability and efficiency. As we dive deeper into this guide, we will explore the syntax, best practices, and potential pitfalls of using switch case in Java, ensuring you have a solid grasp of this essential programming concept.
Whether you are a beginner eager to learn Java or an experienced developer looking to refine your skills, understanding the switch case statement will provide you with a valuable tool for writing clean and effective code. In this article, we will cover everything you need to know about using switch case in Java, including its benefits, limitations, and practical examples to help you master this important concept.
What is a Switch Case in Java?
The switch case in Java is a control statement that allows a variable to be tested for equality against a list of values, known as cases. This statement can be used as an alternative to multiple if-else statements, making the code easier to read and maintain. When a matching case is found, the corresponding block of code is executed.
How Does the Switch Case Work?
To effectively use the switch case in Java, you begin by defining a variable that you want to evaluate. The switch statement follows, specifying the variable to be checked. Each case is defined with a specific value, followed by a colon, and the code to be executed if that case matches the variable. The switch case structure also includes a default case, which acts as a fallback if none of the specified cases match.
What Are the Benefits of Using Switch Case in Java?
- Improved Readability: Switch case makes it easier to read and understand complex decision-making processes.
- Efficiency: It can lead to better performance compared to multiple if-else statements, especially with numerous conditions.
- Cleaner Code: The switch case structure allows for a more organized approach to coding, reducing clutter.
- Ease of Maintenance: Adding or modifying cases is simpler and less error-prone.
What Are the Limitations of Switch Case in Java?
While the switch case statement is a powerful feature, it does have limitations. One of the primary restrictions is that the variable being evaluated must be of a specific data type. In Java, switch case can only handle primitive types such as integers, characters, and strings. Additionally, the switch statement does not support ranges or conditions, which might require additional logic using if-else statements.
How to Implement Switch Case in Java?
To implement the switch case in Java, follow these steps:
- Define a variable to be evaluated.
- Use the switch statement followed by the variable in parentheses.
- Define each case with a value, followed by a colon.
- Implement the code block for each case.
- Optionally include a default case to handle unmatched conditions.
Can You Provide a Simple Example of Switch Case in Java?
Certainly! Here is a simple example of how to use the switch case statement in Java:
public class SwitchCaseExample { public static void main(String[] args) { int day = 3; String dayName; switch (day) { case 1: dayName ="Monday"; break; case 2: dayName ="Tuesday"; break; case 3: dayName ="Wednesday"; break; case 4: dayName ="Thursday"; break; case 5: dayName ="Friday"; break; case 6: dayName ="Saturday"; break; case 7: dayName ="Sunday"; break; default: dayName ="Invalid day"; break; } System.out.println("Day " + day + " is " + dayName); } }
What Are the Best Practices for Using Switch Case in Java?
To maximize the effectiveness of the switch case in Java, consider the following best practices:
- Use break Statements: Always include break statements to prevent fall-through behavior unless explicitly desired.
- Use Default Case: Always include a default case to handle unexpected values and improve code robustness.
- Keep Cases Simple: Avoid overly complex logic within cases; if necessary, consider using separate methods.
- Limit the Number of Cases: For a large number of cases, consider using other structures such as maps or lists for better organization.
How to Debug Switch Case in Java?
Debugging switch case statements can be straightforward if you follow certain steps. Here are some strategies to effectively debug switch case in Java:
- Print Statements: Use print statements to display the value of the variable being switched on and the corresponding case being executed.
- Check Data Types: Ensure that the variable and case values are of compatible data types to avoid mismatches.
- Test Edge Cases: Test your switch case with various inputs, including boundary cases and invalid values.
Conclusion: Why is the Switch Case Statement Important in Java?
In conclusion, the switch case statement in Java is an invaluable tool for developers, enabling cleaner, more organized code for decision-making processes. By understanding its syntax, benefits, limitations, and practical applications, you can harness the power of switch case effectively in your Java projects. Whether you are building simple applications or complex systems, mastering the switch case will significantly enhance your programming skills and code efficiency.
Article Recommendations
- Best True Story Movies
- Efficient Strategies_0.xml
- Brand Building_0.xml
- How Did Jennifer Syme Pass Away
- Arianna Lima 2024
- How To Make Raphael In Infinite Craft
- Madison Beer Nude Leak
- Deacon Johnson
- Bryan Hearne
- Mia Hamm Soccer Player


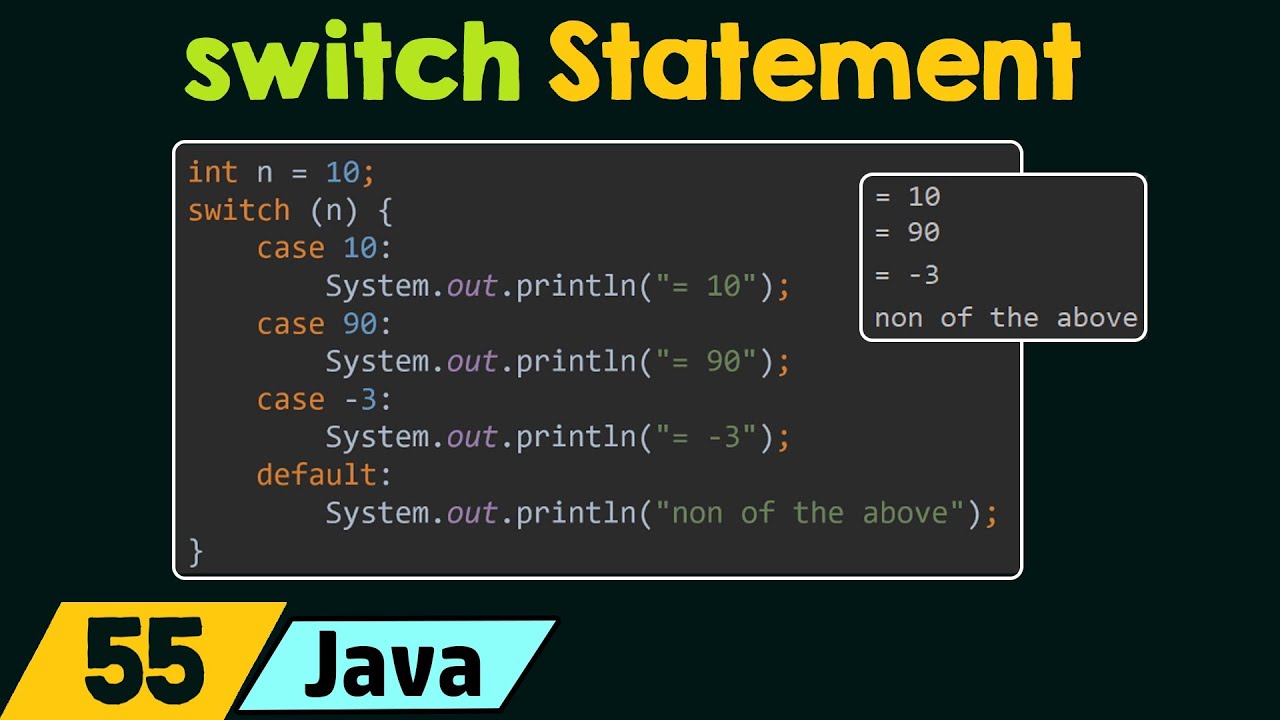